Table of contents
Reading time: 14 minutes
To put it figuratively, all modern sites are based on the “three pillars” - HTML, CSS and JavaScript. HTML is responsible for the skeleton of a web page, CSS - for its shell, and JavaScript gives the elements on the page dynamism. But of the three listed above, only JS is formally considered a full-fledged programming language. It has variables, classes, functions and other attributes that rely on it. Therefore, JavaScript is a mandatory and indispensable tool for a webmaster.
Why JavaScript is needed
JS is primarily responsible for the user's interaction with the page. Scripts in this language define what happens if the user clicks on a certain button or enters data into an interactive form. In addition, JavaScript can:
- show popups;
- pre-check user data before sending it to the server;
- refresh the page in the background via AJAX;
- work with cookies;
- manipulate html tags and attributes.
Basic knowledge of html is useful not only for webmasters, but also for other professionals:
- Internet marketers and seo-optimizers to independently add ready-made scripts to the site and find possible errors in the code;
- business analysts to audit websites;
- to all owners of Internet resources in order to monitor the state of the site and promptly make changes (or contact programmers).
Language Benefits
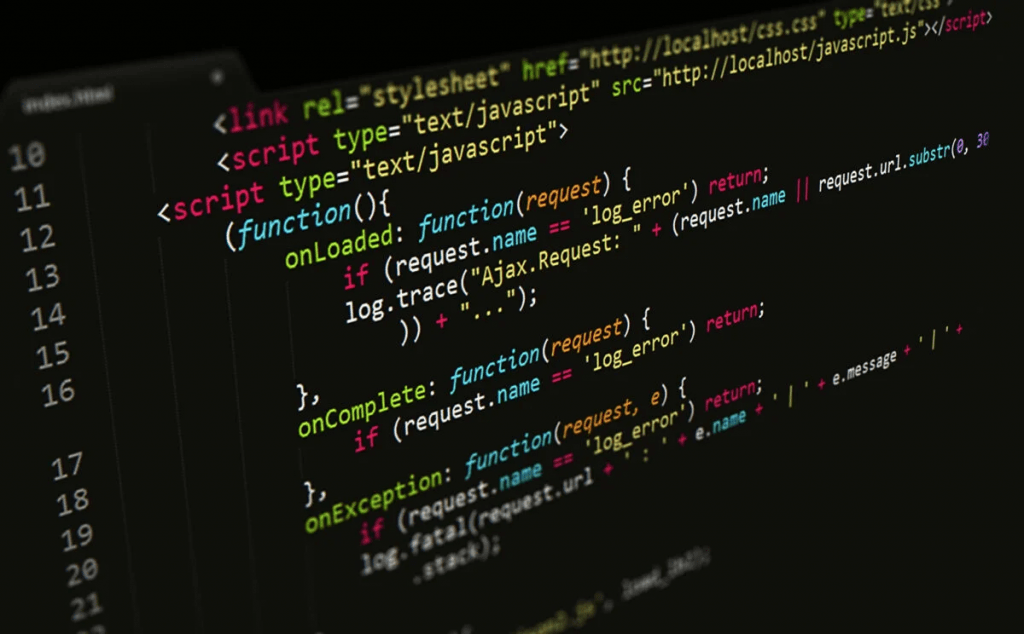
- Versatility of use.
JavaScript is easily interpreted by all browsers. The result of interpretation is platform independent. Java script sites look the same in Google Chrome, Safari and Opera.
- Clear logic.
Commands in JS are closer to normal human language than machine code. Most tasks in JavaScript are solved concisely, in just a few lines.
- Data loading speed.
JavaScript processing is partly done by the client (browser). This reduces server load and speeds up page loading.
- Frameworks and extensions.
JS developers have access to many ready-made solutions - libraries and frameworks - that speed up the creation of sites and simplify complex tasks.
- Wide functionality.
Java script covers most of the needs of frontend and backend web development. It can be used to ensure the operation of interactive functions both on a small landing page and on a complex corporate portal.
Along with the advantages of JS, there are also a few of its disadvantages.
- Limited tabbed browsing.
For security reasons, JS does not have access to third-party tabs. Its effect is limited to the current page.
- Unable to upload files directly.
JavaScript itself cannot read and process files through a browser. This, again, is dictated by security requirements. Working with third-party objects is supported only by the Node.js extension.
Differences between Java and JavaScript
Despite their names, JavaScript and the Java general-purpose programming language are very different things. The naming confusion arose when the "progenitor" of JavaScript, the LiveScript language, was planned to be associated with Java. As a result, the languages were never merged, but they did not rename JavaScript back either.
JS is more associated with web development. Although there are now tools like Node.js that take JavaScript outside of the browser, the language is still more commonly used to build websites. Java is a more versatile tool. You can quickly and conveniently develop full-fledged desktop programs on it.
How it works and basic commands
In web development, JavaScript is inextricably linked to html. To insert a JS script into a page, the <script> pair tag is used. The src attribute specifies the path to the script if it is stored in an external file.
Example:
<script src="/path/to/script.js"></script>
For scripts stored in the current folder, a short path is sufficient.
<script src="script.js"></script>
Small scripts can be inserted directly into the body of the page instead of being moved to external files. Now let's look at a few basic JS commands for data input/output.
Alert
The Alert statement shows the programmed message to the user and an interactive OK button. To demonstrate a window with a welcome inscription, let's create such a small file in .html format and open it through a browser.
<!DOCTYPE HTML>
<html>
<body>
<script>
alert('Hello world!');
</script>
</body>
</html>
Result.

Usually, Alert is tied to some event on the site - a button click, data entry, time since the start of the session, etc.
Confirm
A command used to confirm an action on the current page. Confirm displays a window with a message and two options - "OK" and "Cancel". Depending on the user's choice, the server receives the message "true" or "false" and executes further conditions.
This is what the base of the team looks like.
<!DOCTYPE HTML>
<html>
<body>
<script>
confirm( 'Subscribe to our useful newsletter');
</script>
</body>
</html>
Message box in the browser.

Prompt
The operator calls up a dialog box for entering data. The input field can be filled with an example placeholder.
All information from the user received through the Prompt function is sent to the server. In the future, the transferred data can be used to perform internal tasks on the site.
<!DOCTYPE HTML>
<html>
<body>
<script>
prompt("How can I contact you?", "Enter your name");
</script>
</body>
</html>
This is what the example code looks like.

How variables work in JavaScript
One of the foundations of any object-oriented programming language is variables. JavaScript is no exception.
JS has both mutable and immutable variables. The first are set through the var operator. If we write the string var x = 1, x will be assigned a value of one. But, if within the same block (function or cluster) the command var x = 3 is entered in the code, the x value will be rewritten to three. Thus, if you accidentally or intentionally re-assign a value to the code, an error can break through. In bulky parts of the program, the developer may simply not keep track of the variable name.
To prevent overwriting in advance, you should use the let statement. Consider the difference with examples.
var greeter = "Hello new user";
var times = 2;
if (times > 1) {
var greeter = "Hi Friend";
}
console.log(greeter);
In this case, the value of the variable greater, which displays the welcome message, depends entirely on the value of the variable times. But we can also write an independent output.
let greeting = "Hi Friend";
if (true) {
let greeting = "Welcome back";
console log(greeting);
}
console.log(greeting);
Here we output two separate phrases. In this case, the first one is always shown, and the second - only when the condition is met. Variables are named the same and do not conflict with each other.
The above example is simple, and in general does not cause problems. But often the redeclaration of a variable is difficult to track down, and this becomes a critical error. Choosing the right parameter helps prevent such cases.
JavaScript Frameworks
Programmers rarely write the code themselves from the first to the last line. To perform typical tasks, there are standardized frameworks. Thanks to them, the code is clean and safe, and development does not last for months. Consider the most common products for JavaScript in practice.
React.js
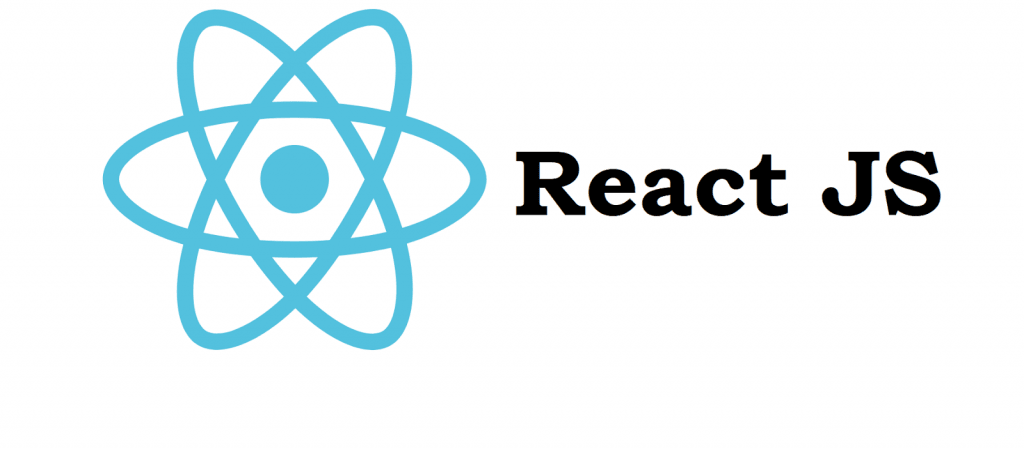
According to the results of many surveys, this is the most used framework in JS development. First of all, React is designed to create complex user UI interfaces. One of its most useful features is the component structure. A component is a single block in a design that is reusable. The second important feature of React is the ability to easily bind JS and HTML using JXS technology. Due to JXS, page design becomes as dynamic and flexible as possible.
It's also worth noting that React is easy to learn for beginners, thanks to its easy debugging and large user community.
Vue.js
A framework that can be easily integrated into ready-made code. Vue's transition and animation effects add beauty to a site without being overwhelming or slowing down page loads. Like React, Vue supports JXS calls and modular increments, making it a handy frontend tool. The framework command line (CLI) allows developers to quickly assemble ready-made blocks.
Svelte
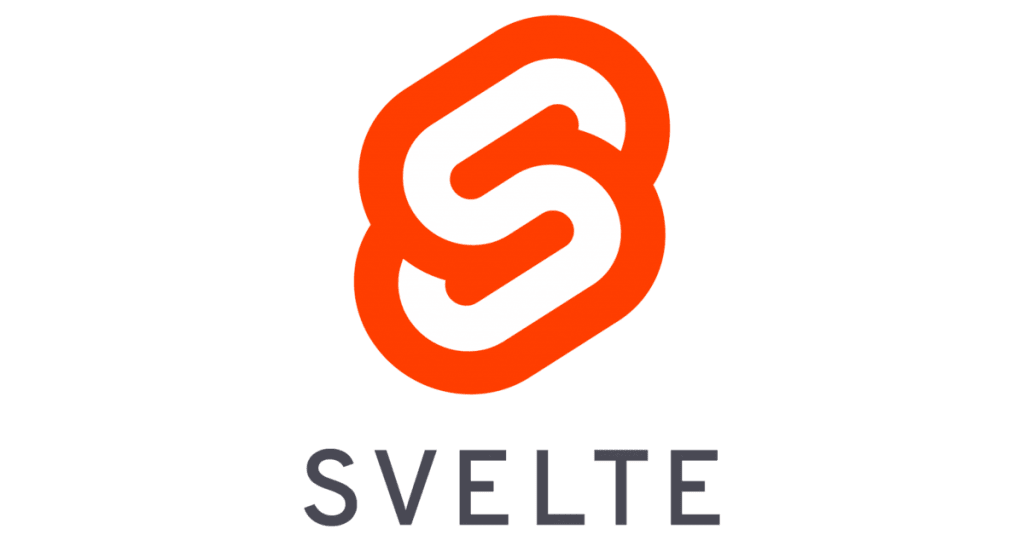
One of the fastest frameworks to develop. Svelte processes the code directly, without referring to the Document Object Module, due to which it achieves high speed results. In addition, Svelte has a simplified syntax. Teams on it take up less space. The performance of the framework is also on top.
Angular
Another component framework. The product is developed by Google, and it also provides support. An important “trick” of Angular is a well-thought-out mechanism of dependencies between classes, modules, functions and components. It helps keep the code clean and minimize bugs. Thanks to the structure on Angular, you can create not only websites, but also complex corporate applications.
Aurelia
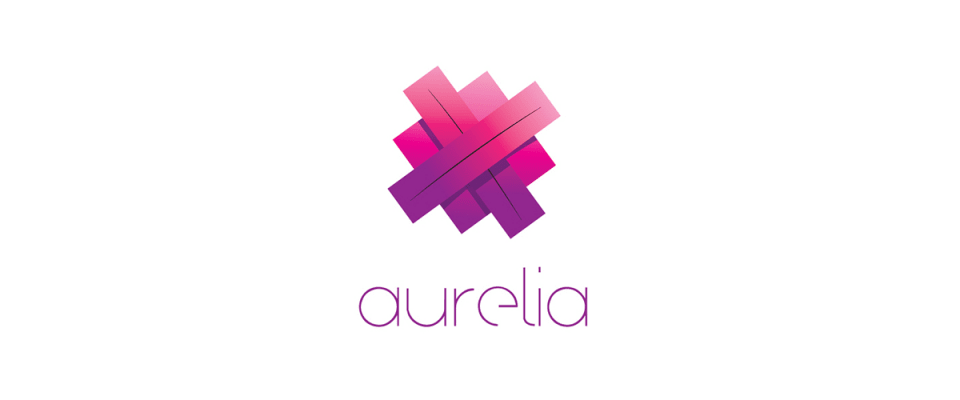
A powerful memory-efficient framework. Due to its standardization, it easily integrates with other JS tools - for example, JQuery and Bootstrap.
Ember.js
This framework provides an internal tool for testing the written code, thereby reducing the likelihood of errors. Basically, Ember works on the basis of templates, which allow you to flexibly customize HTML and other data formats. Another advantage of the framework is the provision of secure authentication for sites and web applications.
Backbone.js
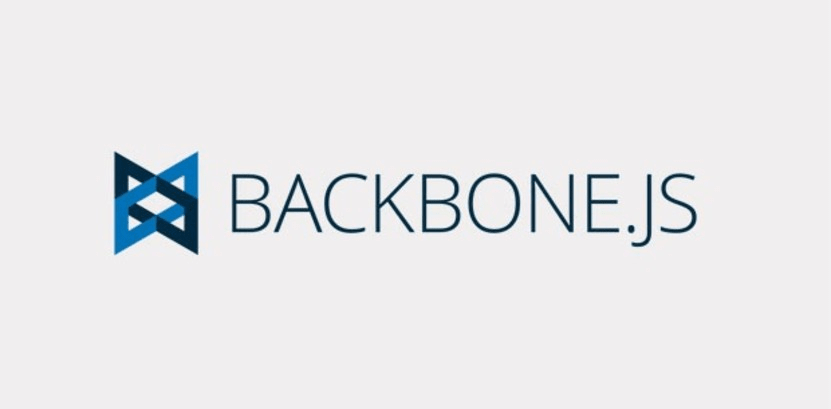
A tool containing over 100 JavaScript extensions. Backbone code is compatible with all browsers, including mobile ones. Functions and modules on this framework look compact and “clean”, which greatly simplifies development.
Conclusion
JavaScript is an object-oriented programming language, a versatile web development tool. It is used to add dynamism to site elements, to display pop-up windows, handle cookies, and perform other tasks. Development in JavaScript is most often carried out on frameworks - libraries that extend the capabilities of the language and speed up the creation of software. Tools like Node.js take JavaScript outside of the browser and allow you to develop independent desktop apps for desktop and mobile devices.