Table of contents
Reading time: 3 minutes
Good afternoon everyone. This article will be about how to debug an app/game on Android.
Alternative ready-made solutions:
- The Android SDK includes a set of debugging tools. The most important debugging tool is LogCat. The android.util.Log class allows you to categorize messages based on importance.
- You can find a lot of applications for catching logs on the Play Market, but 90% of them require Root rights. What is not safe for us.
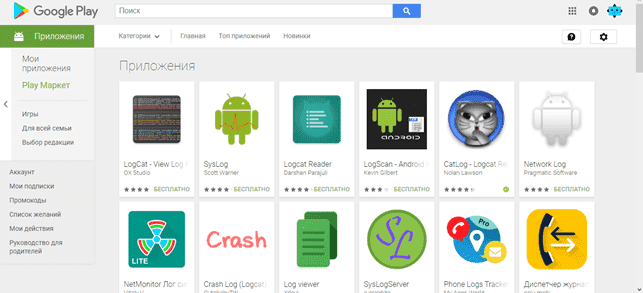
I myself often encountered a situation where I needed to know about the state of the application at the moment, to know the result of functions and the result of conditional statements. There were no ready-made solutions. Therefore, in the absence of a large amount of time, it was decided to implement the first idea that appeared in the brain, which was already tired of crutches.
Solution #1 (don't do this)
Create a Text element on the Canvas. We stretch this element across the entire width of the screen and we will display the entire log in this textbox. I once needed this solution for debugging when integrating AdColony AppLovin advertising networks.
The console example was used in only one script (AdsManager) and did not require external calls. Since the script itself had a singleton - Instance, the Text element could in theory be called from anywhere. But you shouldn't do it. Since the value of the Text variable can be null.
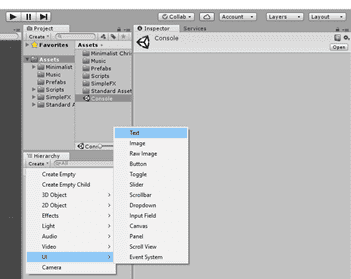
Fig - 1. Create a text element
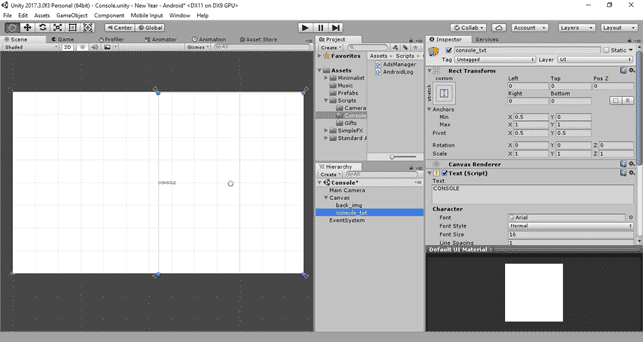
Fig - 2. Placing the text element
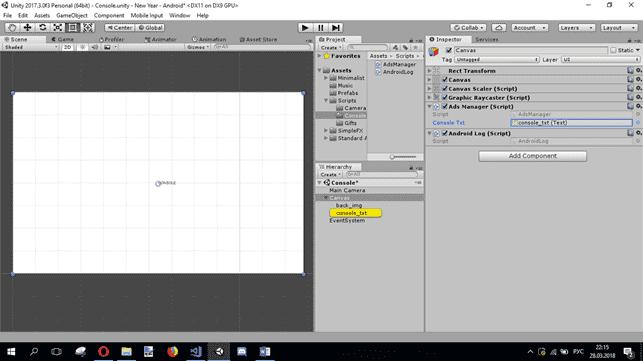
Fig - 3. Assigning a variable to the script in the inspector
Code example
using UnityEngine;
using UnityEngine.UI;
public class AdsManager : MonoBehavior {
public static AdsManager Instance;
void Awake() {
Instance = this;
}
public Text consoleTxt;
void Start() {
consoleTxt.text = "";
for (int i = 0; i < 10; i++) {
LogToConsole(System.Math.Pow(Random.Range(0,1000),i));
}
}
private void LogToConsole(object log) {
consoleTxt.text += "\n" + log.ToString();
}
}
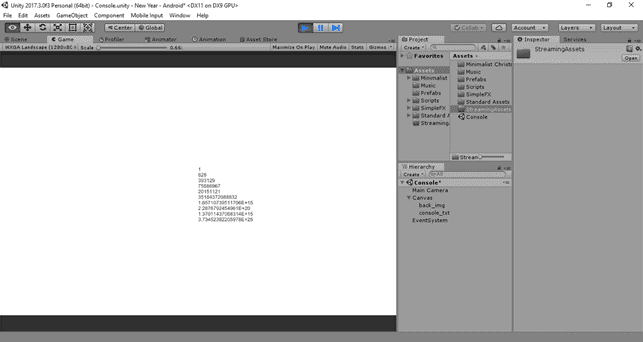
Fig - 4. The result of the work
Conclusion: the solution is not optimal. You cannot see the full log, since the text field is not infinite and there is a limit to everything. The second reason is inconvenient output and reading the log. Of course, it would be possible to get confused and make the scrolling panel appear at the click of a button, BUT the game is not worth the candle.
Solution #2 (optimal)
The solution is as simple as daylight. We will output the entire log with LOG ERROR WARNING marks to the test file. The file can be found in the application's source path or in Streaming Assets. The file is overwritten after each launch of the application. Also, for convenience, after recording, you can display the time from the moment the application was launched to the moment of the log.
Android file path:
.../Android/data/com.YourCompany.ProjectName/files/log.txt
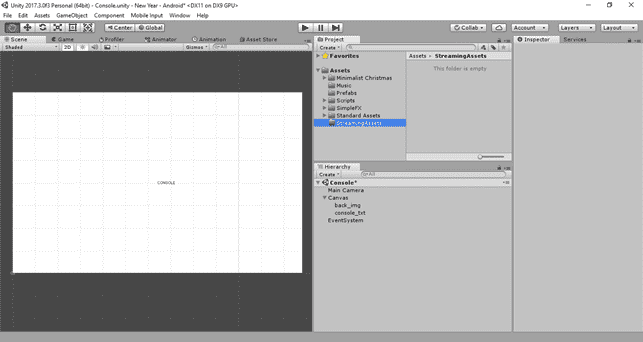
Fig - 5. Create the StreamingAssets folder otherwise we will get an error about the missing path in the editor
Important, there are different paths for writing a file for the editor and Android.
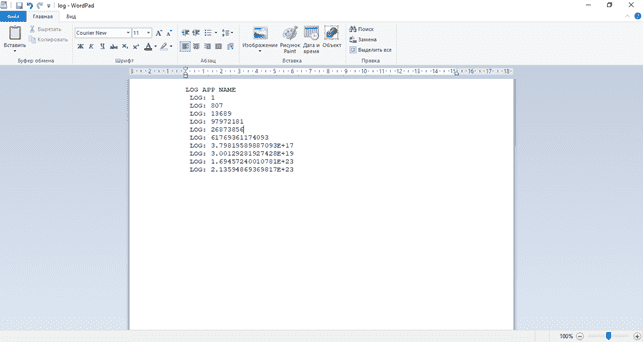
Fig - 6. Result for Win10
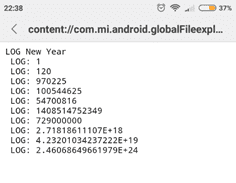
Fig -7. The result of the work for Android
Code example
using UnityEngine;
using System.IO;
public class AndroidLog : MonoBehavior {
static string pathFile = "";
void Start() {
#if UNITY_EDITOR
pathFile = Application.dataPath + "/StreamingAssets//log.txt";
#endif
#if UNITY_ANDROID && !UNITY_EDITOR
pathFile = Application.persistentDataPath+"//log.txt";
#endif
if (File.Exists(pathFile)) File.WriteAllText(pathFile, "LOG " + Application.productName);
else File.Create(pathFile);
}
public static void LogComment(object str) {
File.AppendAllText(pathFile,"\nLOG: " + str.ToString());
}
public static void LogError(object str) {
File.AppendAllText(pathFile, "\nERROR: " + str.ToString());
}
public static void LogWarning(object str) {
File.AppendAllText(pathFile,"\nWARNING: " + str.ToString());
}
}
Output: The solution is good because it is cross-platform.
The quality of the log, readability has noticeably improved, and you can get a detailed log.